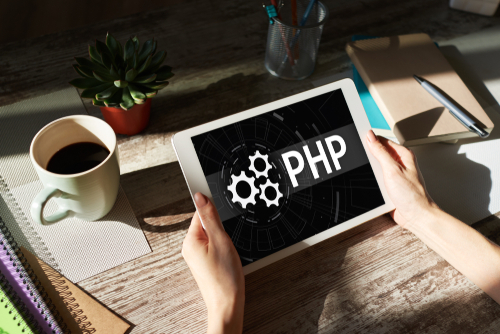
With all the computer and scripting languages available, one measure of success is the number of tools and resources created for a specific language. PHP is one such server-side scripting language, primarily used for Web development, and which drives many millions of websites, either with custom PHP code or using Frameworks built in PHP. The language has a wealth of tools and resources. Here are a selection of 50 tools and resources in various categories that are worth checking out.
Notes: Due to space limitations , this is by no means comprehensive of coverage of all categories but rather a broad starting point of PHP tool and resource references to build upon. All tools and references listed here are free unless noted, but may have other fees associated with their use.
PHP Installations and IDEs
Before you code in PHP, you need an installation with a Web server and database, as well as an editor or IDE (Integrated Development Environment).
- XAMPP — XAMPP (*nix Apache + MySQL +PHP +Perl) is a ‘LAMP stack” distribution that is ideal for local Web testing for PHP on Windows, Mac OS X and Linux. One alternative is MAMP for Mac and Windows, although Linus (“L”) support is missing.
- NetBeans IDE — The popular NetBeans IDE might be better known for Java development but is also suitable for developing PHP code.
- Eclipse PHP setup — Eclipse is an open source IDE for many languages, with support for PHP as part of the Eclipse PHP Development Tools package. One alternative is Aptana Studio, which is based on Eclipse. Some items in this list may have plugin versions for Eclipse and/or Aptana.
Learning PHP
There are thousands of tutorials, online books and resource sites on PHP. Here are a few with which to get started.
- PHP.net Manual — Anything you need to know about PHP syntax and functions can be found in the PHP.net Manual, along with usage examples.
- PHPBuilder.com — PHPBuilder is a PHP resource site with a collection of articles, tutorials, tips, code library, forum and more.
- Zend Developer Zone — Zend Developer Zone is a collection of news, tutorials and forums for PHP. Check out PHP 101: PHP for the Absolute Beginner if you are just starting out.
- PHP Freaks — PHP Freaks has tutorials, blogs, news, IRC chat, forums and more for PHP.
- PHP: The Right Way — PHP: The Right Way offers to teach new developers PHP best practices, and is or will be available in nearly 20 languages.
- PHP Academy — PHP Academy offers web development courses for free, including videos.
- WikiBooks — Wikibooks has a online PHP book aimed at beginners, that walks you through installation, the basic of the language and advanced use.
- Killer PHP — Killer PHP offers Web development tutorials, including video, focused around PHP.
Code Banks and Libraries
Extending what comes with PHP is easy with numerous code banks and libraries.
- SimplePie — SimplePie is an RSS/Atom feed parser that allows you to incorporate feeds into your PHP Web site.
- PHP Classes — PHP Classes is a collection of PHP scripts, tutorials, book reviews, forums and more.
- PHPExcel — PHPExcel can read, write or even create Excel spreadsheets for use on a Web site.
- The League of Extraordinary Packages — PHP League is a collection of useful, tested PHP packages that are distributed via Packagist and Composer.
- SwiftMailer — SwiftMailer is a PHP 5 MIME-compliant email library that supports not just SMTP, sendmail and postfix but also custom Transports, and can handle large attachments and inline images.
- PHP-CPP — PHP-CPP is a library for developing very fast PHP extensions in C++.
- PHP.net Image Processsing and GD — This is the portion of the PHP.net manual to get you started on image processing capabilities in PHP. Also look into MagickWand for PHP and PHP Image Workshop.
- GeoCoder — If you’re building geo-aware Web applications, Geocoder PHP offers an abstraction layer for geocoding, has support for over two dozen third-party services including Google Maps, Bing Maps, OpenStreetMap, MapQuest, TomTom, ArcGIS Online and more, and has subprojects for Symfony2, Laravel and other frameworks.
- PHPMailer — PHPMailer is a popular library for sending email from PHP and is used by CMSes such as Drupal and Joomla!
Templating Engines
The likelihood that you are using PHP for developing Web sites (as opposed to command line use is high). As such, you are very likely generating Web pages dynamically, and a good templating engine will make that easier.
- Smarty — Smarty is one of the early templating engines, and has the philosophy that includes an easy syntax that does not require PHP knowledge, and clean separation between code (PHP) and presentation (HTML/CSS).
- Twig — Twig – created by the Symfony framework creator – markets itself as a “modern” template engine for PHP, using more concise, template-oriented syntax, with shortcuts for common patterns.
- Savant 3 — Savant is an object-oriented template system that uses PHP language and can be extended and custom-compiled.
HTTP and REST APIs
Need to write PHP code that consumes content from the Web, or to allow that from your site? This section is for you.
- Requests for PHP — Requests for PHP is based on the Python library of the same name and is used as a more structured layer over cURL and fsockopen, to produce and consume HTTP requests.
- Guzzle — Guzzle is another HTTP requests client that helps eliminate having to work directly with cURL and also stream contexts and sockets.
- Apigility — Apigility is a REST API development tool that also speaks RPC and offers features that include versioning, normalization, validation, authentication (several flavors) and documentation of APIs.
Development Tools
This category includes Package and Dependency Management, Version Control, Build and Deployment, Testing, Analyzing, Debugging and Documentation Tools and anything else that helps with the fine-tuning and management of code.
- Composer Dependency Manager for PHP — Composer is a popular dependency manager for PHP packages that manages them on a per-project basis rather than globally.
- Packagist — Packagist is a PHP package archivist that aggregates Composer-installable packages.
- Git — Git may not be as mature as Subversion (svn) but it’s said to be faster, more flexible, and is a version control system of choice for many tech companies and developers. Atlassian has a comprehensive set of Git tutorials to get you started.
- Subversion — Originally a Tigris.org project, now transitioning to Apache, Subversion is one of the more mature code version control systems.
- Phing — Phing is a PHP project build tool based on Apache Ant, and which can run PHPUnit and SimpleTest unit tests.
- Xinc — Xinc is a continuous integration system and control server that works with Phing and Subversion.
- phpDox — PhpDox documentation generator uses PHP-Parser by default for the codebase info collection, which is then stored in XML format.
- Faker — When you don’t have data to test, Faker (inspired by similar libraries for Perl and Ruby) can generate it for you. If you also need mock objects, method stubs, etc., check out Phake.
- XDebug PHP profiling — XDebug is a PHP debugger and profiler, with WebGrind as a frontend.
- PEAR — PEAR (PHP Extension and Application Repository) is a framework for distributing/ installing reusable PHP components.
PHP Frameworks
PHP frameworks give you the scaffolding to develop large Web applications which will need to be scaled at a later point.
- Laravel 4 — Laravel bills itself as “the PHP framework for Web artisans” and includes the Blade templating system.
- Silex — Silex is a PHP microframework built on Symfony 2 Components.
- CakePHP — CakePHP is yet another popular PHP framework but requires no configuration file (just a database), is MVC-bsaed, and has a set of security and validation tools plus more.
- CodeIgniter — CodeIgniter is a full PHP framework with a small footprint and follows Agile methodology.
- phpMyFAQ — PhpMyFAQ is open source software for creating and managing FAQs (Frequently Asked Questions) pages (free if copyright notice maintained).
- Zend Framework — Zend Framework is by the same people that offer the PHP scripting engine.
- Symfony — Symfony is both a PHP Web framework and a set of nearly 30 standalone reusable PHP components.
- Phalcon — Phalcon is a C extension-based framework that claims to be the fastest, beating out even CodeIgniter, Kohana, Laravel and Zend.
- FuelPHP — FuelPHP is a hierarchical MVC framework with ViewModels, and which supports routing using PHP closures.
- Slim — Slim is a micro-framework for fast development of PHP applications and APIs.
PHP-Based Content Management Systems
Don’t feel like building a Web site or application from scratch? There are numerous PHP-based CMSes with vibrant community support and hundreds or even thousands of plugins and page themes. Or you can write custom code to extend a CMS yourself.
- Drupal — The open source Drupal CMS may not be quite as popular as WordPress but it’s up there, and is built on the Symfony framework.
- Joomla! — Joomla! is another CMS amongst the most popular (they claim being the most popular based on community size), with millions of sites running, including corporate sites.
- WordPress — This is the self-hosted version of the popular WordPress.com, and one of the most popular CMSes if not the most, with thousands of free and paid plugins, themes and tutorials, and millions of Web sites running.